Using the AM2320 Temperature and Humidity Sensor with ESP-Wroom 32
Introduction
In this tutorial, you will learn how to use an AM2320 temperature and humidity sensor with an ESP32 board. The AM2320 is a simple to use, high-performance sensor that can measure temperature and humidity over a long distance up to 20 meters. It has high accuracy and stability and can be used in various applications such as HVAC, dehumidifiers, weather stations, home appliances, etc. The sensor communicates with the ESP32 using either a single-wire or an I2C interface.
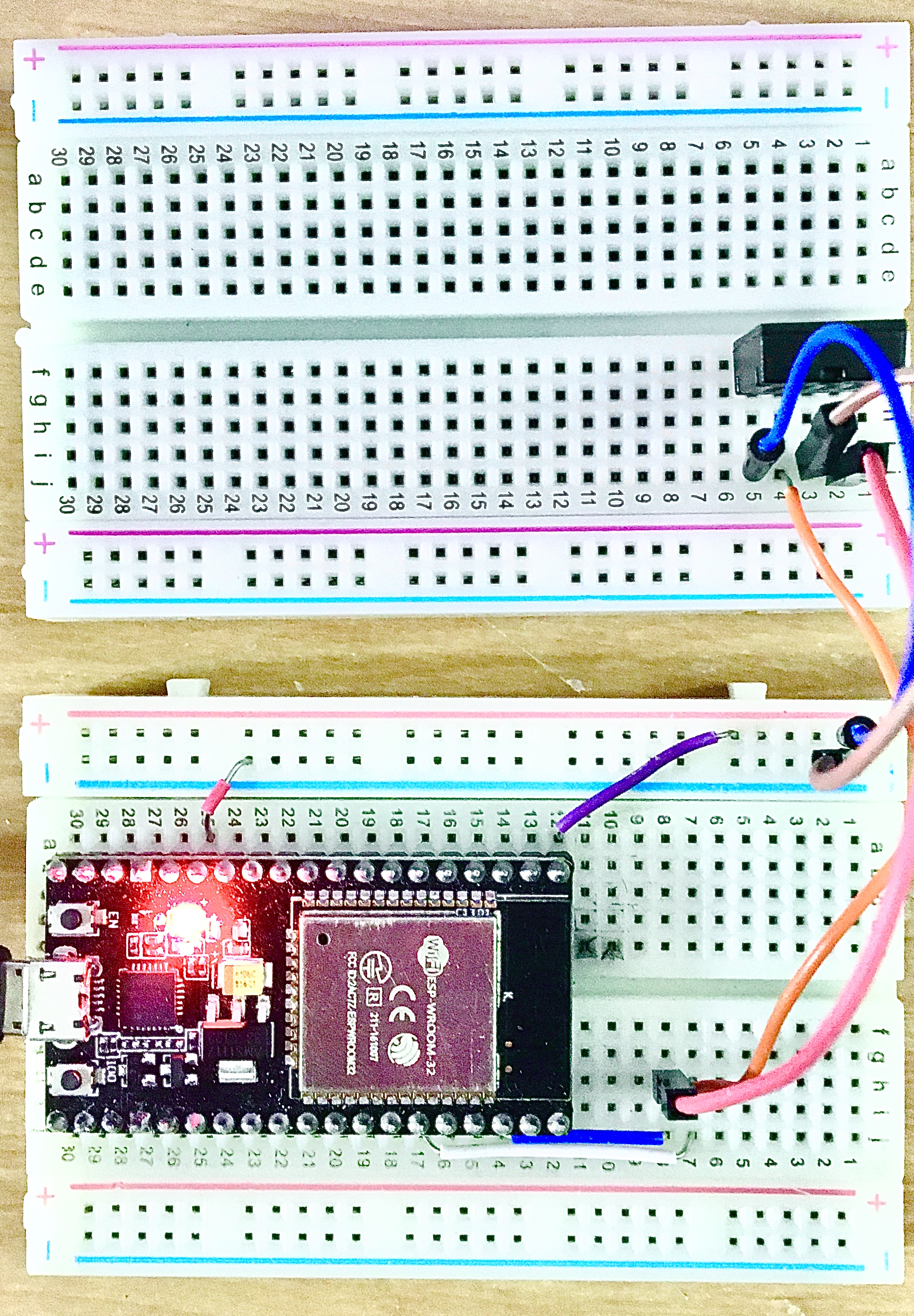
Materials
To follow this tutorial, you will need the following materials:
Wiring and Setup
The wiring and setup of the AM2320 sensor and the ESP32 board depend on the communication mode you choose: single-wire or I2C. In this tutorial, we will use the I2C mode, which requires two resistors as pull-up for the SDA and SCL lines²³. The wiring diagram is shown below:
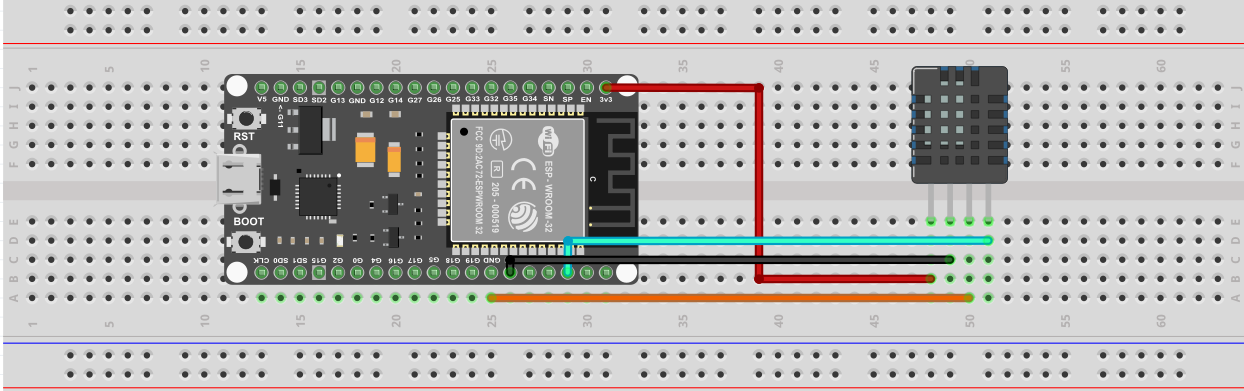
Below are the pinouts for each module:
Step 1: Wiring Connections
The AM2320 temperature sensor is connected to an ESP-WROOM-32 microcontroller using the following pin configuration:
AM2320 Temperature Sensor | ESP-WROOM-32 Pin |
---|---|
VCC | 3.3V |
GND | GND |
SDA | GPIO21 (SDA) |
SCL | GPIO22 (SCL) |
To set up the Arduino IDE for the ESP32 board, you need to follow these steps:
Step 2: Install Board
In order to interact with the ESP Wroom 32 Using the Arduino IDE, you must install the following board:
- ESP32 By Espressif (Within IDE)
Click here for instructions how to install boards within the arduino application.
Step 3: Install Libraries
The libraries that are used are:
- Adafruit_Sensor (ZIP file, Unavailable Within IDE)
- Adafruit AM2320 Sensor Library (Within IDE)
Click here for instructions to install libraries within the arudino application, and here to add library through ZIP file.
Full Code
The full code for reading temperature and humidity from the AM2320 sensor and printing them to the serial monitor is shown below. You can copy and paste it into your Arduino IDE and upload it to your ESP32 board.
#include "Adafruit_Sensor.h"
#include "Adafruit_AM2320.h"
Adafruit_AM2320 am2320 = Adafruit_AM2320();
void setup() {
Serial.begin(9600);
while (!Serial) {
delay(10); // hang out until serial port opens
}
am2320.begin();
}
void loop() {
Serial.print("Temp: ");
Serial.print(am2320.readTemperature());
Serial.print(" C");
Serial.print("\t\t");
Serial.print("Humidity: ");
Serial.print(am2320.readHumidity());
Serial.println(" \%");
delay(2000);
}
Programming & Logic
Let's go through the logic of the code line by line:
- These lines include the necessary libraries for using the Adafruit sensor and AM2320 temperature and humidity sensor.
#include "Adafruit_Sensor.h"
#include "Adafruit_AM2320.h"
- Here, an instance of the Adafruit_AM2320 class is created and named am2320. This object will be used to interact with the sensor.
Adafruit_AM2320 am2320 = Adafruit_AM2320();
- The setup() function is called once at the start of the program. In this function:
- Serial.begin(9600); initializes the serial communication with a baud rate of 9600 bits per second.
- The while loop is used to wait until the serial port is opened before proceeding.
- am2320.begin(); initializes the AM2320 sensor.
void setup() {
Serial.begin(9600);
while (!Serial) {
delay(10); // hang out until serial port opens
}
am2320.begin();
}
- The loop() function is called repeatedly after the setup() function. In this function:
-
Serial.print("Temp: "); and Serial.print("Humidity: "); are used to print descriptive labels for the temperature and humidity readings.
-
Serial.print(am2320.readTemperature()); and Serial.print(am2320.readHumidity()); retrieve the temperature and humidity readings from the AM2320 sensor, respectively, and print them using the Serial.print() function.
-
Serial.print(" C"); and Serial.println(" %"); are used to print the units of temperature (Celsius) and humidity (%), respectively.
-
delay(2000); pauses the program for 2000 milliseconds (2 seconds) before repeating the loop.
void loop() {
Serial.print("Temp: ");
Serial.print(am2320.readTemperature());
Serial.print(" C");
Serial.print("\t\t");
Serial.print("Humidity: ");
Serial.print(am2320.readHumidity());
Serial.println(" \%");
delay(2000);
}
Troubleshooting
Hardware Tips
-
Make sure the AM2320 sensor is properly connected to your Arduino board. Double-check the wiring to ensure the correct pins are connected between the sensor and the Arduino.
-
Ensure that your Arduino board has the necessary I2C communication capabilities. Most Arduino boards have built-in I2C support, but if you're using a different board or module, make sure it supports I2C communication.
-
Provide stable and regulated power supply to the Arduino board and the sensor. Fluctuations in power can affect the sensor readings and the stability of the communication.
Software Tips
-
Confirm that you have installed the required libraries, namely
Wire
andAM2320
, in your Arduino IDE. You can install them by going to "Sketch" -> "Include Library" -> "Manage Libraries" and searching for the library names. -
Check if you have the correct versions of the libraries installed. Incompatibilities between library versions can cause errors or unexpected behavior.
-
Before uploading the code to your Arduino board, ensure that the correct board and port are selected in the Arduino IDE under "Tools" -> "Board" and "Tools" -> "Port," respectively.
-
If you encounter issues with the sensor readings or communication, consider adding additional debugging statements to the code to print intermediate values or error codes. This can help you identify the problem more effectively.
-
If you plan to use the code in a larger project, consider implementing error handling and recovery mechanisms in case of sensor failures or communication errors. This could involve retrying the sensor reading, implementing timeouts, or providing feedback to the user.
Output
If everything works as expected, you should see something like this on your serial monitor :
Temp: 25.3 C Humidity: 54.8 %
Temp: 25.4 C Humidity: 54.7 %
Temp: 25.5 C Humidity: 54.6 %
Temp: 25.4 C Humidity: 54.5 %
...
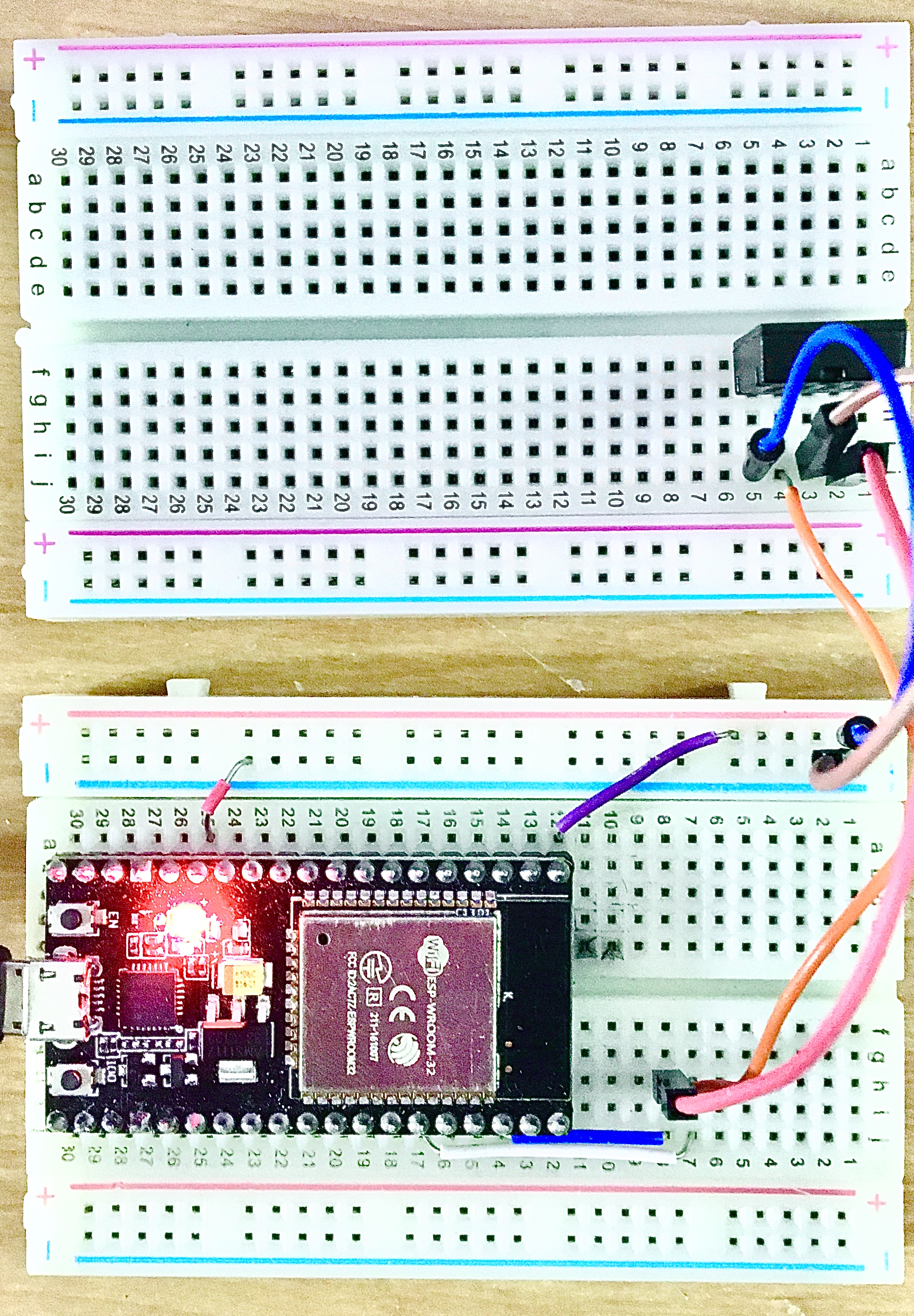
Conclusion
In this guide, you learned how to use an AM2320 temperature and humidity sensor with an ESP32 board in I2C mode. You learned how to wire and set up the sensor and the board, how to write and upload code to read data from the sensor, how to explain the programming logic of the code, how to troubleshoot potential issues, and how to view the output on the serial monitor. You can use this knowledge to create your own projects that involve temperature and humidity sensing with ESP32