16. DS1307 RTC Module
An RTC module is a highly useful component in projects that require precise timekeeping. Unlike your Arduino's built-in timer, which resets every time the power is cycled, an RTC module retains the correct time and date even when powered down, thanks to its onboard battery backup.
Materials
Component | Image |
---|---|
Breadboard | ![]() |
Jumper wires | ![]() |
Arduino Uno R4 Minima | ![]() |
DS1307 RTC Module | ![]() |
Instructions
- Make the following connections using the breadboard and jumper wires.
Connections
- GND to GND
- VCC to 5V
- SDA to SDA
- SCL to SCL
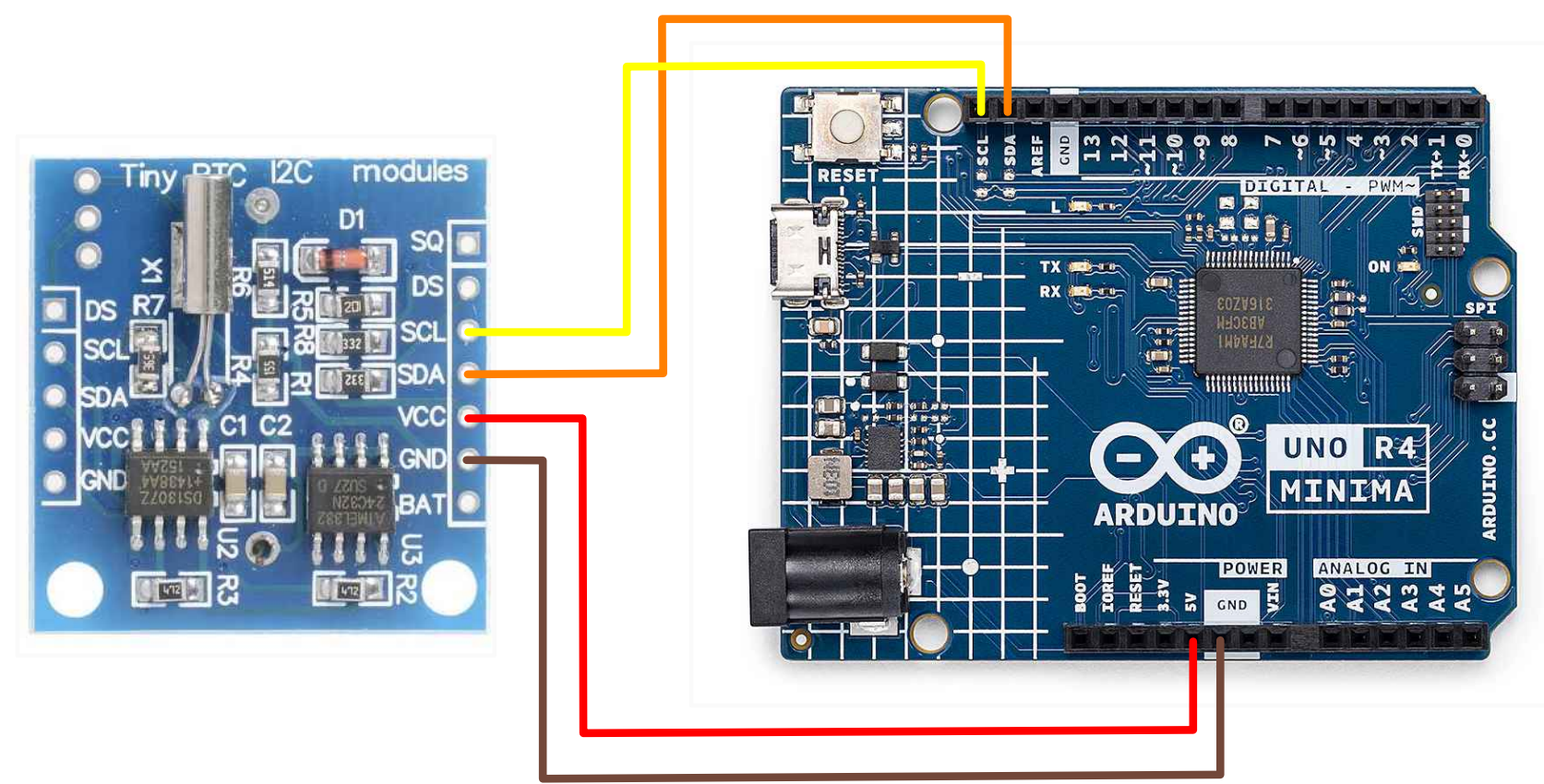
-
Download the "RTClib" library from the Library Manager in the Arduino IDE.
-
Paste the following code into your main Arduino sketch:
Code
// Importing the necessary libraries
#include <Wire.h>
#include <RTClib.h>
RTC_DS1307 rtc;
void setup() {
// setting the serial monitor to print the output
Serial.begin(9600);
Wire.begin();
if (!rtc.begin()) {
Serial.println("Couldn't find RTC");
while (1);
}
if (!rtc.isrunning()) {
Serial.println("RTC is NOT running, let's set the time!");
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
}
}
void loop() {
DateTime now = rtc.now();
// printing out the present date and time
Serial.print(now.year(), DEC);
Serial.print('/');
Serial.print(now.month(), DEC);
Serial.print('/');
Serial.print(now.day(), DEC);
Serial.print(" ");
Serial.print(now.hour(), DEC);
Serial.print(':');
Serial.print(now.minute(), DEC);
Serial.print(':');
Serial.print(now.second(), DEC);
Serial.println();
// print every second
delay(1000);
}
-
Connect your Arduino to your laptop using a USB-C cable and upload the code to the arduino.
-
Test! Observe the serial monitor print out the date and time on the serial monitor.
Prev | Next |
---|---|
15. Water Level Detection Sensor | 17. Sound Sensor |